Potential Issues With Spring Jpa Properties You Should Avoid in Production
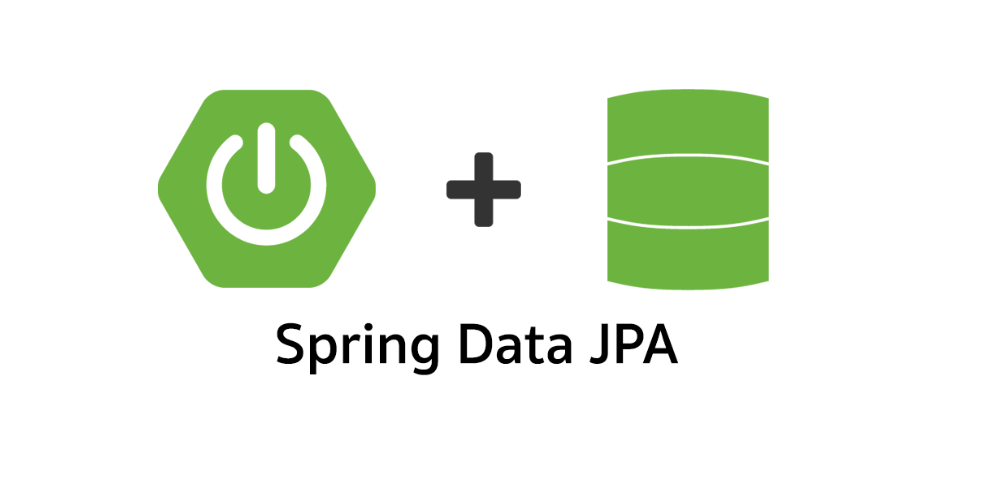
Introduction
Java developers love the Spring Framework since it has gained immense popularity due to its simplicity, flexibility, and robustness. Spring is a powerful tool that simplifies the development of enterprise applications by providing various features and functionalities. One such feature is the Spring Data JPA, which is an abstraction over the JPA specification. Although Spring Boot provides a powerful auto-configuration feature that makes it easy to configure and set up various components of an application, misconfiguring certain properties can lead to unintended consequences and potential issues. In this article, we will focus on two Spring JPA properties, “spring.jpa.hibernate.ddl-auto” and “spring.jpa.generate-ddl,” and discuss the potential issues that can arise from misconfiguring them.
What is Spring JPA Hibernate DDL-Auto?
Spring Data JPA uses Hibernate as its default JPA implementation. Hibernate is a powerful and widely used ORM framework in the Java ecosystem. One of the most critical aspects of any application that interacts with a database is the database schema. The schema defines the structure of the database tables, their relationships, and constraints. In traditional database-driven applications, developers often create the database schema manually using SQL scripts. However, this can be a tedious and error-prone process, especially when dealing with complex database structures.
Spring JPA Hibernate DDL-Auto is a configuration property that allows Spring to automatically generate and manage the database schema. DDL stands for Data Definition Language, and it refers to the SQL commands used to create and modify database structures. When we set the value of spring.jpa.hibernate.ddl-auto to a specific mode, Spring boot automatically generates and executes the necessary DDL statements to create and modify the database schema at startup time.
DDL-Auto Modes
Spring JPA Hibernate DDL-Auto provides several modes that we can set to define how Spring manages the database schema. The available modes are:
- create
- create-drop
- validate
- update
- none
Let’s look at each mode in detail.
create
In the create mode, Spring creates the database schema from scratch at startup time. If the database schema already exists, Spring drops it and creates a new schema. This mode is useful during the development phase when we want to create a clean database structure each time we run the application.
create-drop
In the create-drop mode, Spring creates the database schema at startup time and drops it when the application shuts down. This mode is useful during development and testing when we want to create and destroy the database schema multiple times.
validate
In the validate mode, Spring validates the database schema against the entity classes at startup time. If there is a mismatch between the schema and the entities, Spring throws an exception. This mode is useful in production environments when we want to ensure that the database schema matches the entity classes.
update
In the update mode, Spring updates the database schema to match the entity classes at startup time. This mode adds new tables, columns, and constraints to the existing schema and modifies the schema to match any changes in the entity classes. This mode is useful during development and testing when we want to modify the database schema without dropping the existing data.
none
In the none mode, Spring does not manage the database schema. This mode is useful when we want to manage the database schema manually or when we use an existing schema that we don’t want Spring to modify.
How is the misconfiguration caused problem
For my current project, I have set the Spring JPA as follows:
jpa:
generate-ddl: 'true'
hibernate:
ddl-auto: update
It caused the problem when we deployed the app, the database migration was failed due to some columns were existed already. Even we tried to drop all the tables and schemes, but the issue was still presented.
When I found the root cause is the misconfiguration of Spring JPA. I changed it to:
jpa:
generate-ddl: 'false'
hibernate:
ddl-auto: none
It fixed the issue immediately. However, it may not be a best practise for both dev and production environment. So, we make it into a local profile in the application.yml
.
---
spring:
config:
activate:
on-profile: local
datasource:
readonly:
initialization-mode: always
jpa:
generate-ddl: 'true'
hibernate:
ddl-auto: update
Conclusion
In this article, we explored the Spring JPA Hibernate DDL-Auto configuration property and its modes. Spring JPA Hibernate DDL-Auto provides an easy and efficient way to manage the database schema using ORM techniques. By setting the value of this property, we can control how Spring creates and modifies the database schema at startup time. This feature is particularly useful during the development and testing phases of an application when we want to create and modify the database schema frequently.
A misconfiguration of these properties may cause issues in production environment. So, the best practise for this is to locate them into a local profile.