Unit Testing for Vue Router Global After Hooks
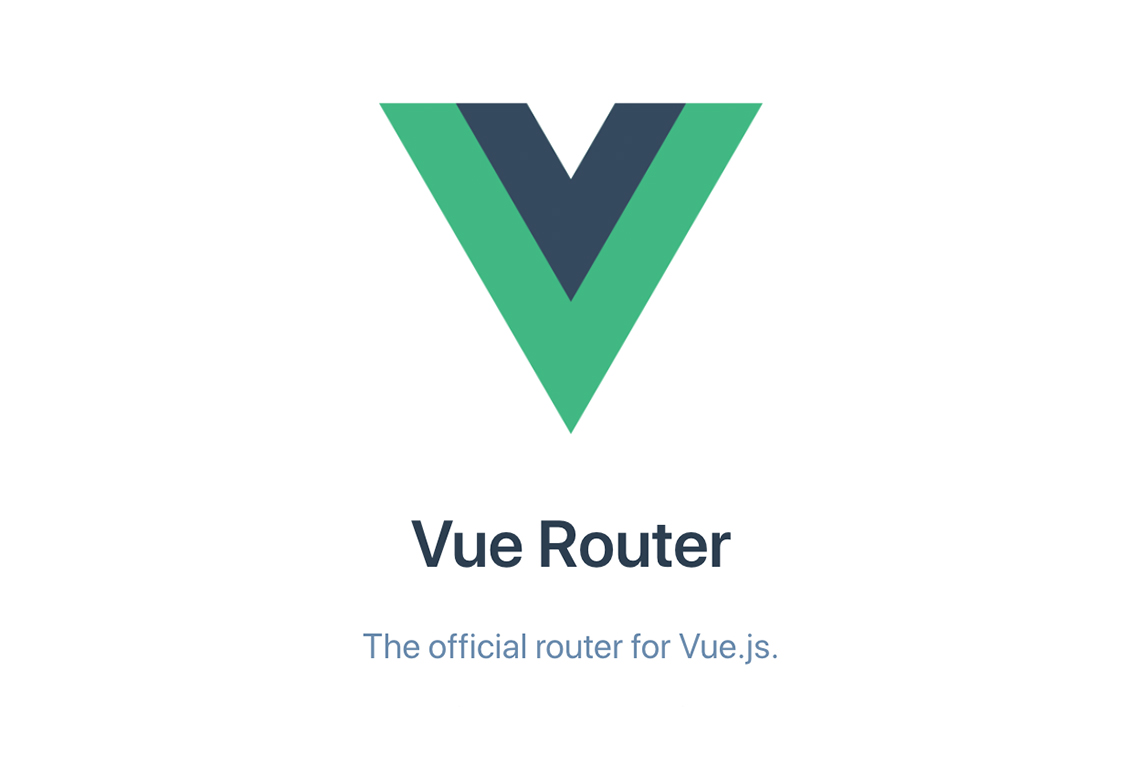
Intro
It is always not easy to do the unit testing for the Vue Router. People prefer to implement end-to-end testing for this. Especially, if you have defined some Vue router global hooks, it would be more difficult to testing the execution of them.
Fortunately, we still can find a way to do the unit testing for the Vue Router Global After Hooks
Background
In my project, I have implemented afterEach
as global after hooks in my Vue router settings. The propose of it is to execute a service call after the user successful login.
The implementation of the code is like this:
router.afterEach( async (to, from) => {
if (from.path === '/callback') {
await JobService.login();
}
});
The code works fine but cause some problem for unit testing. Even we can attempt to call afterEach
by using router.afterHooks[0]()
. We will get an error about we didn’t pass the correct arguments for it.
Refactoring for unit testing
To prepare for unit testing, we may need to refactor the code to make it decoupled. One solution would be independently exporting the afterEach
after hook. We can make the change as below:
{
{
...
router.afterEach((to, from) => afterEach(to, from));
return router;
}
}
// This function is decoupled and independently exported for testing purposes.
export async function afterEach(to, from) {
if (from.path === '/callback') {
await JobService.login();
}
}
After the refactoring, we can do unit testing much easier.
Unit testing
For the unit testing, we can mock the service (JobService), and test if it has been called.
import { afterEach } from "@/router"
import JobService from "@/services/JobService";
jest.mock('@/services/JobService');
describe("when router.afterEach is called from /callback", () => {
it("then JobService.login is called", () => {
const from = { path: "/callback" };
const to = jest.fn();
afterEach(to, from);
expect(JobService.login).toHaveBeenCalled();
})
})
The test will be passed after we execute the test. Cheers!